Practice here the best JavaScript Tricky Interview Questions and Answers.
JavaScript Tricky Interview Questions

Below are the list of Best JavaScript Tricky Interview Questions and Answers
Type coercion is the process of converting value from one type to another (such as string to number, object to boolean, and so on)
By using Number.isInteger() function
In JavaScript, Object Equality is used for two purposes basically. "==" is used for comparing two variables by ignoring its data type whereas "===" is used for comparing two variables by checking its data type.
"===" is used instead of "==" because it can compare two variables by checking data type.
typeof() is a unary operator that returns a string indicating the type of the unevaluated operand whereas instanceof() is a binary operator, accepting an object and a constructor that returns a boolean indicating whether or not the object has the given constructor in its prototype chain.
To delete an element from the javascript array you can use the following technique:
- pop - Removes from the End of an Array.
- shift - Removes from the beginning of an Array.
- splice - removes from a specific Array index.
- filter - allows you to programmatically remove elements from an Array.
You can create an object whose prototype is a given object by the given method i.e. var o = Object. create(Object); it creates a new blank object o whose prototype is the Object function.
To add a number to two variables at once with the help of the following example( foo += -bar + (bar += x)) where x is the number that is added to both variablLinting is the process of running a program that analyses your code for programmatic and stylistic errors.
The steps to get URL and and its parts in JavaScript are:
- window.location.protocol = “http:”
- window.location.host = “css-tricks.com”
- window.location.pathname = “/example/index.html”
- window.location.search = “? s=flexbox”
str.trim() method is used to remove the white spaces from both the ends of the given string.
Syntax:
str.trim()
Return value: This method returns a new string, without any of the leading or the trailing white spaces.
You can use the following code to strip whitespaces from String in JavaScript:
<!DOCTYPE html>
<html>
<head>
<title>
How to remove spaces from
a string using JavaScript?
</title>
</head>
<body>
<h1 style="color: green">
Online Interview Questions
</h1>
<b>
How to remove spaces from
a string using JavaScript?
</b>
<p>
Original string is:
Online Interviews Questions
</p>
<p>
New String is:
<span class="output"></span>
</p>
<button onclick="removeSpaces()">
Remove Spaces
</button>
<script type="text/javascript">
function removeSpaces() {
originalText =
"Online Interviews Questions";
newText =
originalText.replace(/ /g, "");
document.querySelector('.output').textContent
= newText;
}
</script>
</body>
</html>
Output:
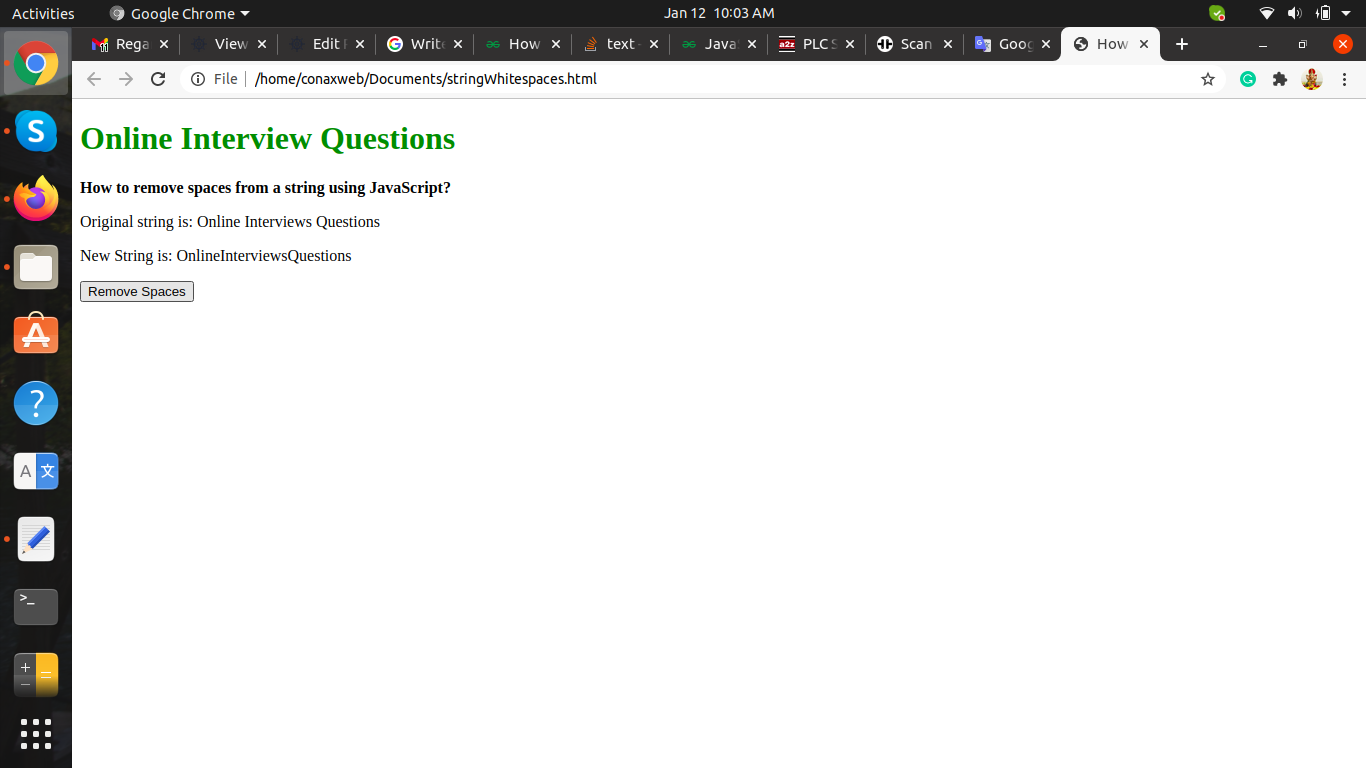
Latest Interview Questions-
Chrome Os Interview Questions
-
CodeIgniter Interview Questions
-
Symfony Interview Questions
-
Laravel Interview Questions
-
PHP String Interview Questions
-
PHP 7 Interview Questions
-
Phalcon Interview Questions
-
PHP Nette Framework Interview Questions
-
Kohana Framework interview questions
-
Fuel PHP Interview Questions
-
Fat Free Framework interview questions
-
Cakephp Interview Questions
-
Aura framework interview questions
-
PHP Interview Questions
-
UML Interview Questions
-
NoSQL Interview Questions
-
Shopify Interview Questions
-
PPC Interview Questions
-
Google Adsense Interview Questions
-
Angular 8 Interview Questions
-
JIRA Interview Questions
-
Bootstrap Interview Questions
-
Apache Tomcat Interview Questions
-
SQLite interview questions
-
Google Adwords Interview Questions
-
jQuery Interview Questions
-
Rest API Interview Questions
-
PHP Array Interview Questions
-
Hive Interview Questions
-
Golang Programming Interview Questions
Subscribe Our NewsLetter
Never Miss an Articles from us.