Handlebars Js Interview Questions
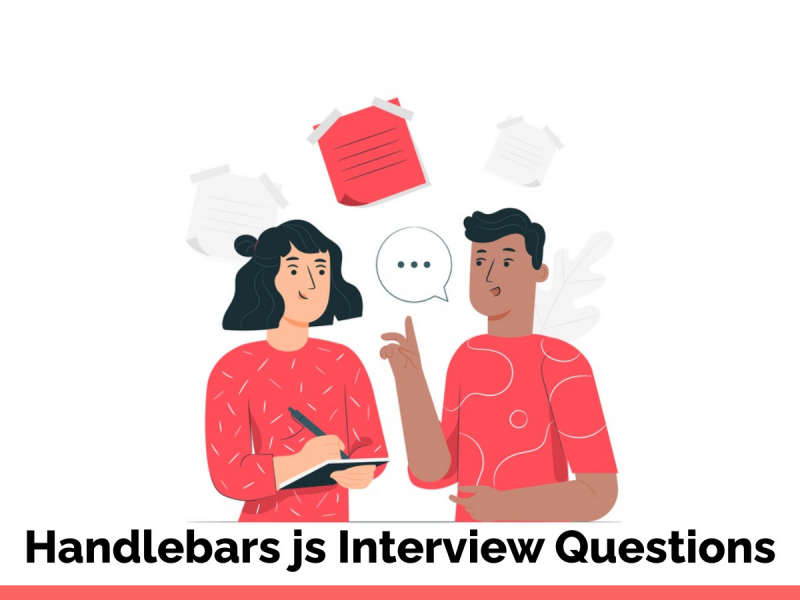
Below are the list of Best Handlebars js Interview Questions and Answers
Handlebar.js is a templating engine that is based on Mustache template language. You can install Handlebar.js by running npm install --save handlebars
command.
4.0.12 is the current stable version of HandlebarsJS.
You can install and configure Handlebar.js using npm or yarn:
npm install handlebars # or yarn add handlebars You can then use Handlebars using require const Handlebars = require("handlebars"); const template = Handlebars.compile("Name: {{name}}"); console.log(template({ name: "Nils" }));
You can configure express-handlebars as our view engine:
app.engine('hbs', exphbs({ defaultLayout: 'main', extname: '.hbs' })); app.set('view engine', 'hbs');
By default, the extension for Handlebars templates is .handlebars. But in the settings here, we've changed it to .hbs via the extreme flag because it's shorter.
Bower is a package manager for the web. It is used to install libraries, assets, and utilities.
You can install bower via npm by running npm install bower
command.
HTML Escaping is defined as the way of Content rendering that uses a double-mustache expression like {{foo}}.
You can use {{!-- {{commented expressions}} --}} synatax for commenting an expressions in Handlebar.js.
Helpers are basically regular functions that take the name of the helper with the helper function as arguments. You can register expression Helper by using the following code:
Handlebars.registerHelper("last", function(array) { return array[array.length - 1]; });
After registration, it can be called anywhere in your templates. Handlebars take the expression's return value and write it into the template.
Partials are a templating concept that is not unique to Handlebars. You can create templates for the reusability purpose. It separates templates into their own file (a Partial), and also uses them in different templates. Partials are just as simple a tool to modularize your templates.
You can register partial in handlebar In the .hbs file containing code by using function Handlebars.registerPartial
Handlebars.registerPartial('myPartial', '{{name}}')
Some built-in helpers are listed below:
- if helper
- Each helper
- Unless helper
- With helper
each Helper:
Each helper is used to iterate over an array. The syntax of the helper is -
{{#each ArrayName}} YourContent {{/each}} . ] } if Helper
The if the helper is similar to an if statement. If the condition evaluates to a truthy value, Handlebars will render the block. We can also specify a template section known as “else section”, by using {{else}}.
The unless helper is the inverse of the if helper. It renders the block when the condition evaluates to a falsy value.
Latest Interview Questions-
Silverlight Interview Questions
-
Entity framework interview questions
-
LINQ Interview Questions
-
MVC Interview Questions
-
ADO.Net Interview Questions
-
VB.Net Interview Questions
-
Microservices Interview Questions
-
Power Bi Interview Questions
-
Core Java Interview Questions
-
Kotlin Interview Questions
-
JavaScript Interview Questions
-
Java collections Interview Questions
-
Automation Testing Interview Questions
-
Vue.js Interview Questions
-
Web Designing Interview Questions
-
PPC Interview Questions
-
Python Interview Questions
-
Objective C Interview Questions
-
Swift Interview Questions
-
Android Interview Questions
-
IOS Interview Questions
-
UI5 interview questions
-
Raspberry Pi Interview Questions
-
IoT Interview Questions
-
HTML Interview Questions
-
Tailwind CSS Interview Questions
-
Flutter Interview Questions
-
IONIC Framework Interview Questions
-
Solidity Interview Questions
-
React Js Interview Questions
Subscribe Our NewsLetter
Never Miss an Articles from us.