Java MVC Interview Questions
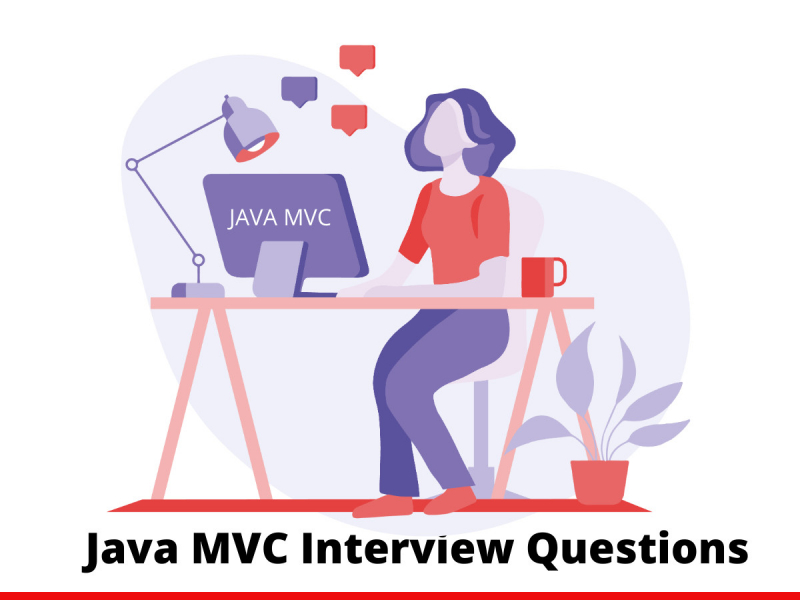
Below are the list of Best Java MVC Interview Questions and Answers
MVC (Model-View-Controller) pattern is an application design model that is comprised of three interconnected parts. Here, the model represents the object. It can also have logic to update the controller when there is a change in data. The view is used to visualize the data. The controller is used to control the data flow into a model object and update the view.
Spring MVC framework is a type of Model-View-Controller architecture that provides components to develop flexible and loosely coupled web applications. This Java framework provides the DispatchServlet class to provide an easy solution to use MVC in the spring framework.
The DispatcherServlet class s used to receive the incoming request and map the request to the right resource like controllers, models, and views. Apart from acting as a controller, the DispatchSetvler is used for error handling, locale resolution, theme resolution, view resolution, and more.
Some of the advantages of using S so you can easily spring MVC is,
- It is a lightweight servlet container so you can easily develop and deploy applications.
- This framework provides powerful configurations for framework and application classes.
- It is easy to inject the test data in the Spring framework using the setter methods.
- It provides flexible mapping functions to easily redirect the page.
- It provides support for fast and parallel development.
- It allows for reusable business code.
The InternalResourcecViewResolver is used to resolve the given URI into the actual URI. It is an implementation of the ViewResolver.
Some of the annotations for HTTP request methods in Spring MVC are,
@RequestMapping - It is used to mark request handler methods.
@RequestBody - It is used to map the body of the HTTP request to an object.
@PathVariable - It is used to bound method argument to a URI template variable.
@RequestParam - It is used for accessing HTTP request parameters.
Some other annotations are
@ResponseBody, @ExceptionHandler, @ResponseStatus, @Controller, @RestController, @ModelAttribute, and @CrossOrigin.
ModelMap is used to pass multiple values from the spring controller to the view. It is dome to render a view. It treats these values as they were within a Map.
ModelAndView is used to pass values to a view that is required by the Spring MVC in one return. It holds both the model and the view. The handler in the MVC returns the object of the ModelAndView and the DispatchServlet resolves the view using the View Resolvers.
The Front Controller design pattern in Spring MVC provides a centralized point for controlling and managing web requests. It handles all the user request and processes the request as per the mapping. This centralized handler can also do authentication, authorization, logging, or tracking of the request and then pass the request to the corresponding handlers.
The Spring MVC Interceptor is used to intercept the requests from the client and handles them. To intercept and process the HTTP request before handing it over to the controller, we use the Spring MVC Interceptor.
It declares three methods based on where to intercept the HTTP request. The three methods are preHandle(), postHandle, and afterCompletion(). These methods provide flexibility in doing all kinds of pre- and post-processing.
The Init Binder is an annotation that is used to customize the request being sent to the controller. It helps to control and format requests that come to the controller as it is defined in the controller. This annotation is used to identify the methods to initialize the WebDataBinder that is used for populating command and form object arguments.
The different types of auto proxies in Spring MVC are,
BeanNameAutoProxyCreator - It automatically creates AOP proxies for beans with the names matching literal values.
DefaultAdvisorAutoProxyCreator - It is a powerful proxy creator that automatically applies eligible advisors in the current context.
AbstractAdvisorAutoProxyCreator - A superclass of DefaultAdvisorAutoProxyCreator which is used to create your own auto proxy creators.
Inversion of Control is a design principle that is used to invert different kinds of controls in object-oriented design. It is used to achieve loose coupling. It inverts the control such as control over the flow of an application, control over the flow of object creation, and binding, etc. With IoC, you can design a loosely coupled class to make it testable, maintainable, and extensible.
The different types of bean scopes available in the Spring MVC are,
- Singleton
- Prototype
- Request
- Session
- Global-Session
Here, the singleton and prototype are the common bean scope available on all Spring application context. Request, session, global session are only available on Web aware context like WebApplicationContext.
A view in the Spring MVC is used to represent the information. It presents the provided information in a particular format. A view page is used to represent the information. The view page is created with a combination of JSP and JSTL. You can also create it using technologies such as Apache Velocity, Thymeleaf, and FreeMarker.
Latest Interview Questions-
CodeIgniter Interview Questions
-
Symfony Interview Questions
-
Laravel Interview Questions
-
PHP String Interview Questions
-
PHP 7 Interview Questions
-
Phalcon Interview Questions
-
PHP Nette Framework Interview Questions
-
Kohana Framework interview questions
-
Fuel PHP Interview Questions
-
Fat Free Framework interview questions
-
Cakephp Interview Questions
-
Aura framework interview questions
-
PHP Interview Questions
-
UML Interview Questions
-
NoSQL Interview Questions
-
Shopify Interview Questions
-
PPC Interview Questions
-
Google Adsense Interview Questions
-
Angular 8 Interview Questions
-
JIRA Interview Questions
-
Bootstrap Interview Questions
-
Apache Tomcat Interview Questions
-
SQLite interview questions
-
Google Adwords Interview Questions
-
jQuery Interview Questions
-
Rest API Interview Questions
-
PHP Array Interview Questions
-
Hive Interview Questions
-
Golang Programming Interview Questions
-
Tailwind CSS Interview Questions
Subscribe Our NewsLetter
Never Miss an Articles from us.